Follow this guide to integrate the SIBS Payment Gateway into your iOS app with our SDK.
Step 1: SDK installation
- Download this file: ios-sibs-stargate-sdk-0.1.1
- Include the SibsSDK.xcframework in the project, as well as the Alamofire dependency:
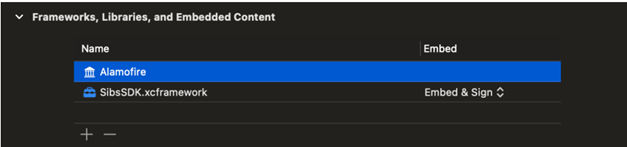
- To use Apple Pay, you need to add and configure this capability in the settings of the App:
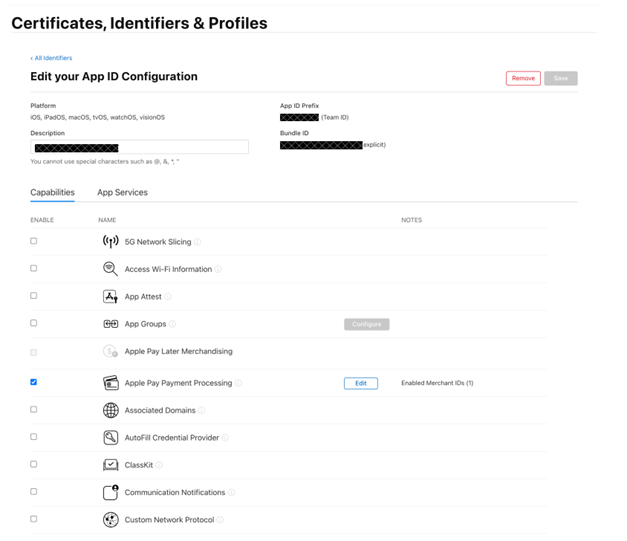
Step 2: SIBS SDK initialization
Initializing the SIBS SDK authenticates you as a merchant. Include the following parameters before sending any further requests.
Parameters
- clientId – client identifier used to authenticate.
- accessToken – access token used to authorise requests.
- environmentSDK – environment in which the SDK will operate.
SDK initialisation method
public init(clientId: String, accessToken: String, environmentSDK: EnvironmentSDK)
Step 3: SDK requests and callbacks
StartPayment
Starts a payment process with the transaction parameters provided.
public func startPayment(from viewController: UIViewController, with data:
TransactionParamsSDK, completion: PaymentResult)
Callback PaymentResult
(Result<TransactionResultSDK, Error>)
GetTransactionStatus
Recovers the status of a previously initiated transaction.
public func getTransactionStatus(transactionID: String, completion: @escaping
TransactionStatusResult)
Callback TransactionResultStatus
(Result<TransactionStatusSDK, Error>)
Data Classes
EnvironmentSDK
public enum EnvironmentSDK{
case quality
case production
}
TransactionParamsSDK
public struct TransactionParamsSDK {
public let terminalID: Int
public let merchantTransactionDescription: String?
public let transactionID: String
public let transactionDescription: String?
public let amount: Double
public let currency: String
public let paymentMethods: [PaymentMethod]
public let client: String?
public let email: String?
public let shopURL: String?
public let shippingAddress: Address?
public let billingAddress: Address?
public let tokenizationParams: TokenizationParams?
public let applePayMerchantId: String?
}
TransactionResultSDK
public struct TransactionResultSDK: Codable {
public let isSuccess: Bool
public let transactionID: String?
public let token: Token?
public let merchant: Merchant?
public let paymentType: String?
public let paymentStatus: PaymentStatus?
public let paymentMethod: String?
public let execution: Execution?
public let returnStatus: ReturnStatus?
}
TransactionStatus
public struct TransactionStatus: Codable {
public let merchant: Merchant
public let transactionID: String
public let paymentType: String
public let paymentStatus: PaymentStatus
public let paymentMethod: String
public let execution: Execution
public let returnStatus: ReturnStatus
public let token: Token?
}